Generate a watch face.
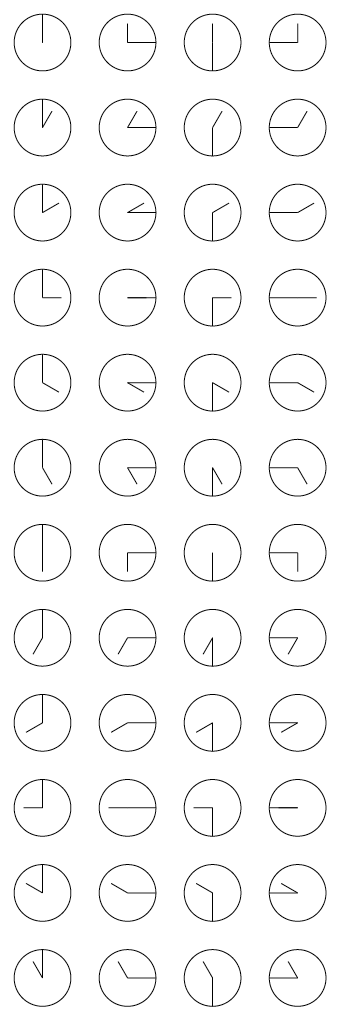
#includepath "~/Documents/;%USERPROFILE%Documents"; // eslint-disable-line
#include "basiljs/bundle/basil.js";// eslint-disable-line
// description
var pw = 120; // for easier handling
var ph = 360; // for easier handling
var gutter = 5; // distance left
var radius = 20; // width and height of the watch face
/**
* the clock function accepts a time returns a clock
* @param {Number} h the hour
* @param {Number} m the minute
* @param {Number} r the radius of the watch face
* @return {Array} an Array of pageItems
*/
function clock (h, m, r){
var angleOff = -90;// we need to offset the lines
var a1 = (m * 6) + angleOff; // clacl the steps for 60 minutes
var a2 = (h * 30) + angleOff; // calc the steps for 12 hours
b.noFill(); // dont want a fill
var circle = b.ellipse(0, 0, r, r); // build the circle
var x1 = b.cos(b.radians(a1)) * (r/2); // x of the big one
var y1 = b.sin(b.radians(a1)) * (r/2); // y
var x2 = b.cos(b.radians(a2)) * (r/3); // x of the small one
var y2 = b.sin(b.radians(a2)) * (r/3); // y
var big = b.line(0,0, x1,y1); // draw the line
var small = b.line(0,0, x2,y2); // draw the line
return [circle, big, small]; // return all that pageItems
}
// main function
function draw(){
b.clear(b.doc()); // clear the current document
b.units(b.MM); // we want to print. use MM instead of default pixels
var doc = b.doc(); // a reference to the current document
// set some preferneces of the document for better handling
doc.documentPreferences.properties = {pageWidth:pw,pageHeight:ph}; // set the page size
doc.viewPreferences.rulerOrigin = RulerOrigin.SPREAD_ORIGIN; // upper left corner
// ----------
// main code goes here
var step = gutter + (radius/2); // the distance
var hour = 0; // the current hour
var minute = 0; // the current minute
var y = step; // init x
var x = step; // int y
var page = b.page(); // get the current page
// loop 12 hours
while(hour < 12){
b.pushMatrix(); // offset the matrix
b.translate(x, y); // to x/y
var c = clock(hour, minute, radius); // draw the clock
b.popMatrix(); // reset the matrix
page.groups.add(c); // create a group
minute +=15; // increase the min
x+= step *2; // increase the x
if(minute === 60){
minute = 0; // reset the minutes
hour+=1; // increase the current hour
y+=step*2; // increase y
x=step; // reset x
} // end if
} // end while
// ----------
// get the filename
var fname = File($.fileName).parent.fsName + '/' + ($.fileName.split('/')[$.fileName.split('/').length - 1]).split('.')[0] + '.indd';
// b.println(fname);
doc.save(fname, false, 'basil', true); // save the file
b.savePNG('out.png'); // save an image
} // end draw
b.go(); // run that thang